Bài viết dưới đây hướng dẫn tạo mã QR (QR code) rất đơn giản bằng PHP trên Debian Linux server.
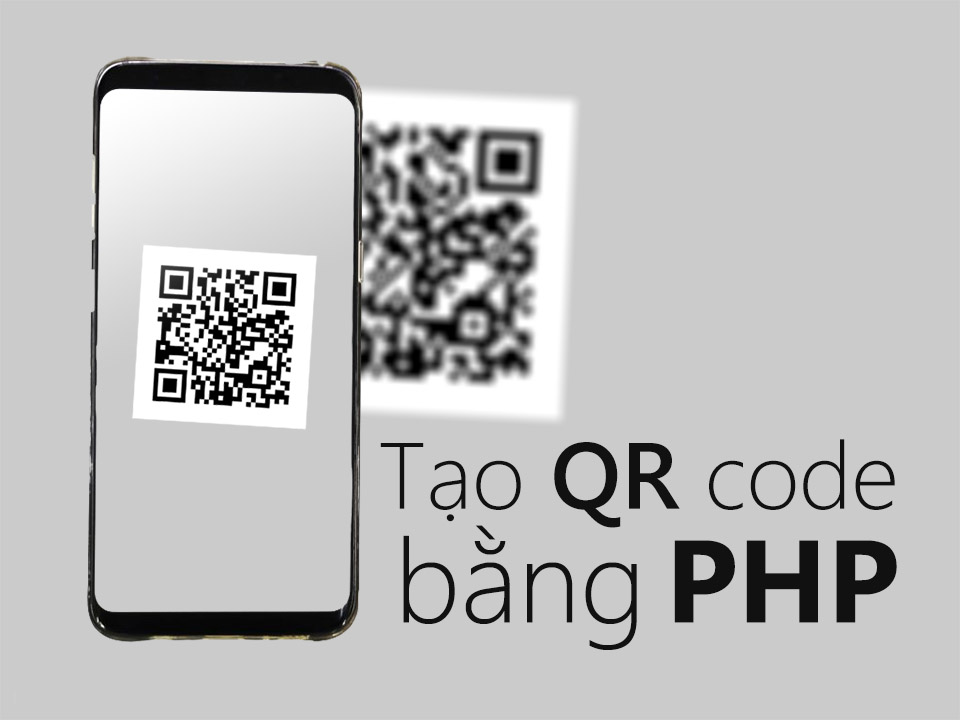
Mục lục
Dùng library tc-lib-barcode
Ta sẽ sử dụng thư viện PHP tc-lib-barcode để tạo mã QR nói riêng hoặc nhiều loại mã vạch khác nói chung.
Chuẩn bị môi trường
Tải về tc-lib-barcode
với composer.
cd ~/public_html mkdir qr cd qr/ composer require tecnickcom/tc-lib-barcode ^1.17
Mã nguồn chương trình
qr/index.php
<?php require_once __DIR__ . '/vendor/autoload.php'; // instantiate the barcode class $barcode = new \Com\Tecnick\Barcode\Barcode(); $content = $_GET['content'] or die('No content'); $type = isset( $_GET['type'] ) ? strtoupper( $_GET['type'] ) : "PNG"; $level = 'H'; // L M Q H $hint = '8B'; // NL=variable, NM=numeric, AN=alphanumeric, 8B=8bit, KJ=KANJI, ST=STRUCTURED $version = '1'; // 1 -> 40 // generate a barcode $bobj = $barcode->getBarcodeObj( "QRCODE,{$level},{$hint},{$version}", // barcode type and additional comma-separated parameters $content, // data string to encode -4, // bar width (use absolute or negative value as multiplication factor) -4, // bar height (use absolute or negative value as multiplication factor) 'black', // foreground color array(-2, -2, -2, -2) // padding (use absolute or negative values as multiplication factors) )->setBackgroundColor('white'); // background color switch ( $type ) { case "PNG": $bobj->getPng(); break; case "SVG": $bobj->getSvg(); break; default: echo "Unsupported type"; } ?>
Dùng Google Chart API
Dù API này đã được ghi nhận là deprecated, tại thời điểm bài viết này được đăng thì tôi thấy vẫn đang hoạt động. Định dạng đầu ra là ảnh PNG. Cú pháp link như sau:
Root URL: https://chart.googleapis.com/chart?
Tham số | Bắt buộc | Mô tả |
---|---|---|
cht=qr | x | Mã QR code. |
chs=<width>x<height> | x | Kích thước ảnh |
chl=<data> | x | Nội dung cần lưu trữ. |
choe=<output_ | UTF-8 [Mặc định]Shift_JIS ISO-8859-1 | |
chld=<error_ | error_correction_level – Mức chịu lỗi margin – Độ dày của đường bao ngoài (mặc định là 4) |
<?php $content = $_GET['content'] or die('No content'); $size = 512; $level = 'H'; $margin = 4; $encoding = 'UTF-8'; $url = "https://chart.apis.google.com/chart?" . http_build_query(array( 'cht' => 'qr', 'chs' => $size . 'x' . $size, 'chld' => $level . '|' . $margin, 'choe' => $encoding, 'chl' => $content )); header("Content-type: image/png"); echo file_get_contents( $url ); ?>
Dùng phần mềm Zint
Zint Barcode Generator là một công cụ tạo mã vạch rất mạnh và portable. Phần mềm dành cho Windows với GUI trên nền tảng Qt hoặc dòng lệnh. Mã nguồn bằng ngôn ngữ C++ biên dịch được cho Linux.
Cài đặt môi trường
Tải mã nguồn Zint về rồi biên dịch bằng GCC.
git clone https://git.code.sf.net/p/zint/code zint cd zint/ mkdir build cd build cmake .. make sudo make install
Mã nguồn PHP gọi tới zint
qr/index.php
<?php $content = $_GET["content"] or die("No content"); $type = isset( $_GET["filetype"] ) ? strtoupper( $_GET["filetype"] ) : "PNG"; $args = array( "--direct", "--barcode=58" /* QR code */ ); $arg_names = array( "filetype" ); foreach ( $arg_names as $name ) { if ( isset( $_GET[$name] ) ) $args[] = empty( $_GET[$name] ) ? "--{$name}" : "--{$name}={$_GET[$name]}"; } switch ($type) { case "PNG": header("Content-type: image/png"); break; case "SVG": header("Content-type: image/svg+xml"); break; default: echo "Unsupported type"; exit; } // Execute qrcode command to generate the QR image data passthru( "zint " . implode( " ", $args ) . " -d '" . addslashes( $content ) . "'", $error ); if ( $error !== 0 ) { $name = "images/invalid.png"; $fp = fopen($name, "rb"); header("Content-type: image/png"); fpassthru($fp); } ?>
Dùng phần mềm qrencode
QREncode phát triển bởi Kentaro Fukuchi được phát triển rất lâu đời (2006), chuyên biệt cho QR code. Vì vậy đây là lựa chọn số 1 cho việc tạo mã QR. QREncode được viết bằng C++ và có thư viện libqrencode
để tích hợp với các app.
Chuẩn bị môi trường
Cài đặt gói qrencode
trên server Debian Linux.
$ sudo apt-get install qrencode
Mã nguồn PHP gọi qrencode
Mã nguồn sau là wrapper bằng PHP cho lệnh qrencode
. Chương trình nhận các cấu hình mã QR từ GET parameter và render ra mã QR dưới nhiều định dạng.
qr/index.php
<?php $content = $_GET['content'] or die('No content'); $type = isset( $_GET['type'] ) ? strtoupper( $_GET['type'] ) : "PNG"; $args = array(); $arg_names = array( 'type', 'size', 'level', 'margin', 'dpi', 'symversion', 'structured', 'kanji', 'micro' ); foreach ( $arg_names as $name ) { if ( isset( $_GET[$name] ) ) $args[] = empty( $_GET[$name] ) ? "--{$name}" : "--{$name}={$_GET[$name]}"; } switch ($type) { case "PNG": header("Content-type: image/png"); break; case "SVG": header("Content-type: image/svg+xml"); break; default: echo "Unsupported type"; exit; } // Execute qrcode command to generate the QR image data passthru( "qrencode " . implode( " ", $args ) . " -o - '" . addslashes( $content ) . "'", $error ); if ( $error !== 0 ) { $name = 'images/invalid.png'; $fp = fopen($name, 'rb'); header("Content-type: image/png"); fpassthru($fp); } ?>
Cách dùng
Các tham số có thể truyền qua GET parameters:
Parameter | Mô tả | Mặc định |
---|---|---|
content | Nội dung được nhúng trong mã QR (bắt buộc) | |
type | Định dạng đầu ra (PNG, EPS, SVG, ANSI, ANSI256, ASCII, ASCIIi, UTF8, ANSIUTF8) | PNG |
size | Kích thước module bằng pixel | 3px |
level | Mức sửa lỗi (L, M, Q, H) | L |
margin | Kích thước đường biên bao ngoài bằng pixel. | 4px |
dpi | Kích thước DPI của ảnh PNG. | 72 |
symversion | Phiên bản QR (1 – 40 với QR, hoặc 1 – 4 với Micro QR) Tham khảo thêm tại ĐÂY | auto |
structured | Tạo structured-append symbol (chia content vào nhiều mã QR), yêu cầu phải chỉ định symversion . Định dạng này không hỗ trợ Micro QR. | false |
kanji | Nội dung có chứa Hán tự. | false |
micro | Mã QR kích thước nhỏ (Micro QR). | false |
Hướng dẫn sử dụng
Ví dụ sử dụng qr/index.php để tạo QR code cho URL bài viết này.
<img src="qr/index.php?content=https://hainh.me/tao-qr-code-bang-php-qrencode" />